Balancing performance optimization with code readability is one of the most common challenges faced by software developers. Code that is optimized for speed and efficiency can become difficult to maintain, while code that prioritizes readability may end up being slow and resource-heavy. Striking a balance between these two goals is key to ensuring long-term success in software development.
In this article, we will explore what performance optimization and code readability mean, how developers often have to trade one off against the other, and most importantly, how to achieve an optimal balance. We’ll also demonstrate practical examples to show how these concepts apply to real-world code.
Table of Contents
1. Understanding Performance Optimization
Performance optimization refers to the process of making code run faster, consume fewer resources, or otherwise become more efficient. This often involves making trade-offs such as using faster algorithms, optimizing memory usage, or minimizing network requests.
Optimizing code can involve reducing time complexity, improving memory efficiency, or minimizing I/O operations. However, blindly focusing on optimization can result in unreadable or unmaintainable code. Let’s look at an example:
Example: Inefficient Code vs. Optimized Code
Inefficient code with O(n²) time complexity:
python:
def find_duplicates(arr):
duplicates = []
for i in range(len(arr)):
for j in range(i + 1, len(arr)):
if arr[i] == arr[j]:
duplicates.append(arr[i])
return duplicates
Optimized code with O(n) time complexity using a set:
python:
def find_duplicates_optimized(arr):
seen = set()
duplicates = set()
for num in arr:
if num in seen:
duplicates.add(num)
else:
seen.add(num)
return list(duplicates)
In this example, the original implementation uses nested loops, resulting in poor performance as the input size grows. The optimized version replaces the inner loop with a set-based approach, reducing the time complexity from O(n²) to O(n), making it much more efficient as the dataset scales.
Read More:– Adaptive Software Development: A Comprehensive Guide
2. The Importance of Code Readability
Code readability is crucial for ensuring that code can be easily understood by other developers, maintained over time, and extended with new features. Readable code follows best practices, such as clear variable names, well-structured logic, and the avoidance of over-complicated tricks that obscure the code’s intent.
Readable code matters because software development is often a collaborative effort. If code is difficult to understand, it can slow down development, increase bugs, and make future updates more complex. Let’s explore this with an example.
Example: Unreadable Optimized Code vs. Readable Code
Unreadable optimized code with complex logic
def f(a)
return set(i for i in a if not (a.count(i)) - 1))
Readable code that clearly explains its purpose:
Readable code with clear variable names and comments
def find_unique_elements(arr):
unique_elements = set()
for item in arr:
if arr.count(item) ==1:
unique_elements.add(item)
return list(set(unique_elements))
The second version of the function is much easier to understand because it uses meaningful variable names and has a clear, logical structure. It prioritizes readability without sacrificing the functionality of the code. Readable code is easier to debug, maintain, and extend.
3. Achieving the Balance: Practical Solutions
When striving to balance performance optimization and code readability, developers must adopt a thoughtful approach that involves writing clean, readable code first and then applying targeted optimizations only where necessary. Below are practical strategies and examples to achieve both.
Step 1: Prioritize Clean Code First
Start by writing clear, readable code that follows best practices, such as using meaningful variable names, modular functions, and including comments where necessary. This ensures that the code is easy to maintain and extend in the future.
Readable Code Example:
#clean, readable function to calculate factorial of a number
def calculate_factorial(n):
"""
This function calculates the factorial of a given number 'n'.
It uses a recursive approach for clarity.
"""
If n==0 or n==1:
return 1
else:
return n* calculate_factorial(n-1)
Here, the code is easy to understand due to the clear function name, docstring, and straightforward logic. This should always be your first step before considering any optimizations.
Step 2: Use Profiling to Identify Performance Bottlenecks
Once your code is clean and readable, use performance profiling tools to identify the areas where optimization is actually needed. Optimizing every part of the code indiscriminately can lead to unnecessary complexity. Profiling helps focus on the critical parts of your application.
Example of Using Python’s `cProfile` Tool:
import cProfile
def main():
calculate_factorial(500)
cProfile.run('main()')
Profiling helps highlight performance bottlenecks, allowing developers to pinpoint areas of the code where optimizations can make a tangible difference.
Step 3: Optimize Performance in Targeted Areas
Once bottlenecks are identified, you can begin to optimize the code in those specific areas without sacrificing readability across the entire codebase. One strategy is to replace inefficient algorithms or data structures with more efficient alternatives. However, always comment and structure the code to maintain its clarity.
Practical Optimization Example:
python:
#Optimized factorial calculation using iterative approach
def calculate_factorial_optimized(n):
Optimized factorial function using iteration instead of recursion. This approach reduces stack overflow risk and improves performance.
result = 1
for i in range(2, n + 1):
result *= i
return result
This version uses iteration instead of recursion, which improves performance by avoiding the overhead of recursive function calls. Yet, the function remains readable thanks to clear variable names, structure, and comments.
Step 4: Leverage Modern Compiler Optimizations and Libraries
Many modern compilers and libraries come with built-in optimizations. For example, Python’s built-in functions are already optimized for performance, and using them can reduce the need for custom optimization.
Example of Using Built-in Functions:
Python:
#using Python's built-in factorial function from the math library
import math
def calculate_factorial_builtin(n):
"""
Use Python's built-in factorial function for optimal performance
"""
return math. factorial(n)
By using built-in libraries like `math. factorial()`, you achieve both readability and performance, since these functions are already optimized and well-documented.
Step 5: Test for Correctness and Maintainability
After optimizing the code, it’s essential to test the correctness and maintainability. Make sure that any performance improvements haven’t introduced bugs or reduced the ability of other developers to work with the code.
Testing the Optimized Code:
Python:
def test_factorial():
#Test cases to ensure correctness
assert calculate_factorial_builtin(5)==120
assert calculate_factorial_builtin(0)==1
assert calculate_factorial_builtin(1)==1
print("All test cases passed!")
#Run the tests
test_factorial()
This test ensures that the optimized function produces the correct results, verifying that the balance between readability and performance hasn’t compromised the code’s correctness.
If you’re seeking solutions for Balancing Performance Optimization with Code Readability, HashStudioz is ready to assist. Our expert team can help you implement the best tools for efficient state management, ensuring your application performs seamlessly. Contact us today to discuss your project and see how we can turn your ideas into reality!
Conclusion
Balancing performance optimization with code readability is an ongoing challenge for developers. The key to navigating this is to avoid premature optimization and focus first on writing clean, understandable code. Once you’ve laid a solid foundation, profiling tools can help identify areas where performance improvements are genuinely needed. From there, targeted optimizations can be applied without compromising clarity.
By leveraging built-in libraries, documenting your logic, and testing the code thoroughly, you ensure both efficiency and maintainability. This balanced approach allows developers to create code that not only runs fast but is also easy to work with, promoting long-term project success and team collaboration.
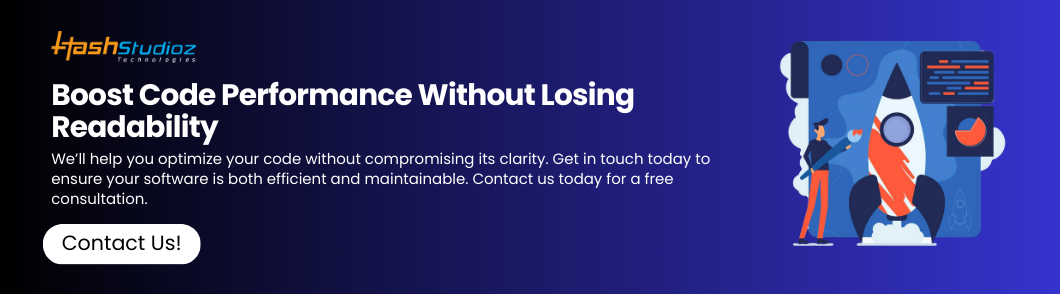